Comparing Strings in Java
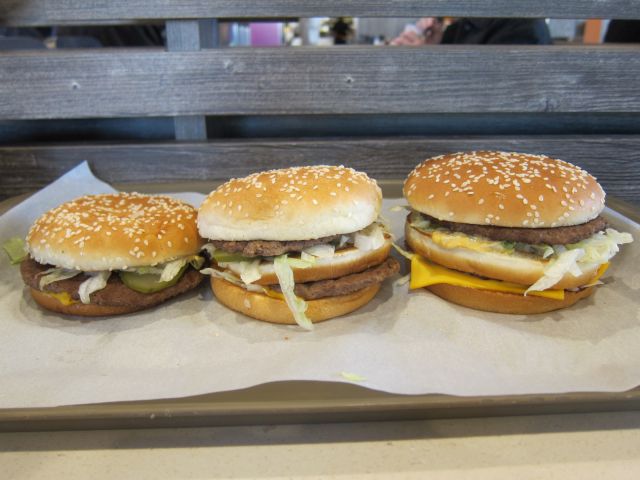
There are three approaches to how to compare strings in Java. Java String CompareTo(), Equals() methods and comparing by reference by == operator. The first two methods compare the string on the basis of content but the last one is by reference.
Compare String in Java by Equals() Method
The String class provides two methods for comparing. See please below.
- public boolean equals(Object object) – compares by value with case sensitivity.
- public boolean equalsIgnoreCase(String object) – compares without case sensitivity by value.
The strings compare are different depending on what method we use, see the example below.
String s1="Stack Throw"; String s2="stack throw"; String s3=new String("Stack Throw"); String s4="Stack Overflow"; System.out.println(s1.equalsIgnoreCase(s2));//true System.out.println(s1.equals(s3));//true System.out.println(s1.equals(s4));//false
Compare String with CompareTo() Method
When we use the method CompareTo() Java analyzes the strings lexicographically and as result, we have three values. Where 0 means that the strings are equal. Values -1 and 1 mean that the strings are different. See the examples below to understand better the returned value of the method.
- s1 == s2 : The method returns 0.
- s1 > s2 : The method returns a positive value.
- s1 < s2 : The method returns a negative value.
String s1="Stack Throw"; String s2="Stack Throw"; String s3="Stack Overflow"; System.out.println(s1.compareTo(s2)); // 0 System.out.println(s1.compareTo(s3)); // -1 (because s1 < s3) System.out.println(s3.compareTo(s1)); // 1 (because s3 > s1 )
Compare String by Using == operator
This operator compares both strings by reference instead of values. To understand it better can help with the following examples.
String s1="Stack Throw"; String s2="Stack Throw"; String s3=new String("Stack Throw"); System.out.println(s1 == s2);//true (both refer to same instance) System.out.println(s1 == s3);//false(s3 refers to instance created in string pool)