Polymorphism in Java
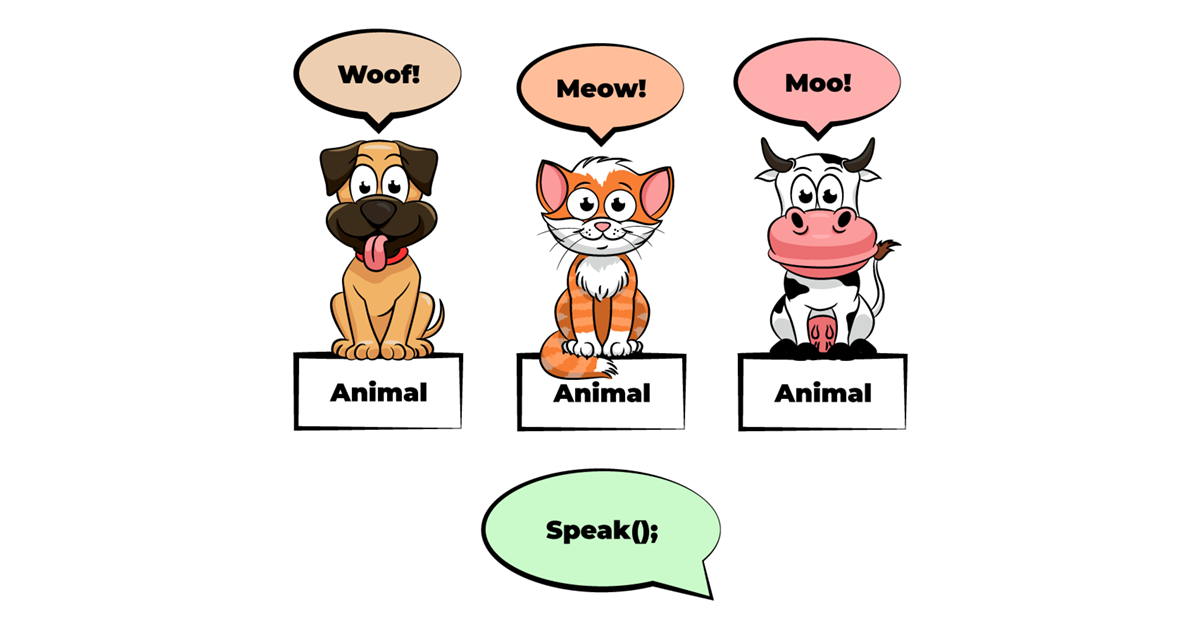
What is Polymorphism
Polymorphism – this term comes from ancient Greek and means “poly” – many, “morphos” – forms. In this case, polymorphism means many forms. Polymorphism is one of the basic foundations of object-oriented programming. You can read about object-oriented programming here.
Polymorphism in Java
Polymorphism in Java is represented comprehensively. There are two forms of polymorphism: compile time and runtime. The language syntax also supports overloading and the ability to rewrite methods.
Java polymorphism involves multiple implementations of one of the interfaces. The code may work very differently, although in general one standard of its description, the interface, will be applied.
Java Polymorphism Example
There is a polymorphism example with a simple implementation.
class Phone { public void call() { makeCall(); // make call } private void makeCall() { System.out.println("make abstract call"); } } class LandLine extends Phone { public void call() { System.out.println("====Land line===="); verifyLine(); // verify wire line findSubscriber(); // find subscriber by the phone number makeCall(); // make call by wire line } private void verifyLine() { System.out.println("verify wire line"); } private void findSubscriber() { System.out.println("find subscriber by the phone number"); } private void makeCall() { System.out.println("make call by wire line"); } } class CellPhone extends Phone { public void call() { System.out.println("====Cell phone===="); verifyCellNetwork(); // verify cell network findSubscriber(); // find subscriber in the network makeCall(); // make cell call } private void verifyCellNetwork() { System.out.println("verify cell network"); } private void findSubscriber() { System.out.println("find subscriber in the network"); } private void makeCall() { System.out.println("make cell call"); } } class Main { public static void main(String[] args) { Phone landLine = new LandLine(); Phone cellPhone = new CellPhone(); landLine.call(); cellPhone.call(); } }
The result would be something like this:
====Land line==== verify wire line find subscriber by the phone number make call by wire line ====Cell phone==== verify cell network find subscriber in the network make cell call
Types of Polymorphism
Polymorphism and programming in Java are closely related to two types of methods: overloaded and overwritten.
Overloaded methods in Java
This example shows how calling a method with the same name can produce different implementations. In our case, depending on the parameters provided, an element on the canvas (point, circle, triangle, or rectangle) will be pseudo-transformed.
class Canvas { public void draw() { System.out.println("Draw dot"); } public void draw(int radius) { System.out.println("Draw circle with radius " + radius); } public void draw(double b, double h) { System.out.println("Draw triangle b=" + b + " h=" + h); } public void draw(int l, int b) { System.out.println("Draw rectangle b=" + b + " l=" + l); } } class Main { public static void main(String[] args) { Canvas canvas = new Canvas(); canvas.draw(); canvas.draw(10); canvas.draw(5.5, 3.4); canvas.draw(7, 3); } }
The result would be something like this:
Draw dot Draw circle with radius 10 Draw triangle b=5.5 h=3.4 Draw rectangle b=3 l=7
Overwriting Methods in Java
When overwriting methods, we must have an ancestor class and an inherited class. Both classes must contain the method to be rewritten later. Consider the example below.
class Phone { public void call() { System.out.println("make abstract call"); } } class CellPhone extends Phone { public void call() { System.out.println("====Cell phone===="); } } class Main { public static void main(String[] args) { Phone cellPhone = new CellPhone(); cellPhone.call(); } }
Result:
====Cell phone====
Static Polymorphism
This type of polymorphism is also called compilation polymorphism. At compile time it can be achieved by overloading methods or overloading operators.
Java does not support operator overloading. Although you can easily overload a method by implementing many such methods with different parameters.
An example of static polymorphism in Java:
public void draw() { System.out.println("Draw dot"); } public void draw(int radius) { System.out.println("Draw circle with radius " + radius); } public void draw(double b, double h) { System.out.println("Draw triangle b=" + b + " h=" + h); } public void draw(int l, int b) { System.out.println("Draw rectangle b=" + b + " l=" + l); }
Runtime Polymorphism
Runtime polymorphism is also called dynamic linkage polymorphism. In this case, methods are overwritten from the class that is inherited.
The example below is a case of upcasting.
class Parent {} class Child extends Parent{} Parent parent = Child();
You can also recall the previously discussed example with the Phone and CellPhone classes. In this case, the call method was overwritten.
Runtime Polymorphism with Inheritance
This example shows the inheritance chain where a car turns into a truck and then into a branded tractor. In all cases, its own drive method will be called.
class Car { public void drive() { System.out.println("Car driving"); } } class Truck extends Car { public void drive() { System.out.println("Truck driving"); } } class VolvoTrack extends Truck { public void drive() { System.out.println("VolvoTruck driving"); } } class Main { public static void main(String[] args) { Car car = new Car(); car.drive(); Car truck = new Truck(); truck.drive(); Car volvoTrack = new VolvoTrack(); volvoTrack.drive(); } }
Result:
Car driving
Truck driving
VolvoTruck driving
Polymorphism in Subtypes
A good example with overloading the + operator. In this case, either string gluing or sum counting occurs.
String s = "hello "; String s1 = "world"; int x=10; int y=22; System.out.println(s+s1); System.out.println(x+y);
Result;
hello world
32
Problems with Polymorphism
- Rather difficult to implement
- Reduces code simplicity
- In some cases, there is an additional load on the system when using polymorphism in a project.
Conclusion
Polymorphism is one of the pillars of OOP that can help make your project architecture more extensible. The code can be reused in such cases, which has a positive impact on the development process and the life cycle of the application.